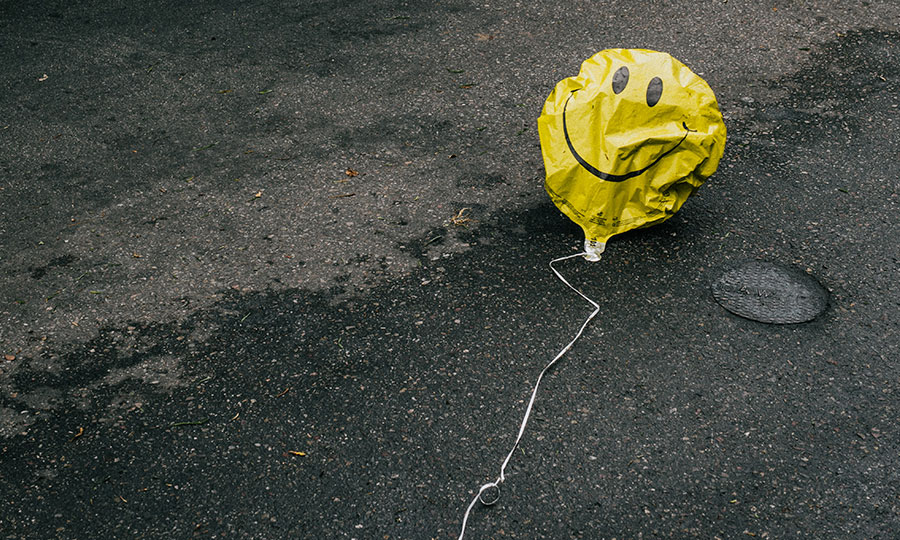
Avoid the cache catastrophe
I feel that no matter what you’re developing you should really be using version control in some way shape or form.
I use BitBucket, mainly because it’s UI is nicer that Github but they both do the same things within the framework of git commands etc.
Myself and my team at Fishtank have all too often made an amend to a website which can include SCSS/CSS or JavaScript and then after the git pull is executed to make the changes live, the changes don’t show.
You can refresh the page as much as you like and – no joy!! … sound familiar…?
You can see the changes looking lovely on your local setup but your client is saying “nothings changed?!?”… sound familiar…?
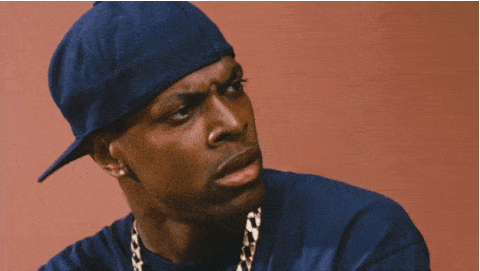
This is normally because its been cached in-browser but a couple of nifty little functions can automatically add the ?v= variable to your enqueued CSS or JS. Forcing browser cache to be flushed based on what version controlled commit you have just put into production.
Here’s how to put an end to all that…
Firstly we add a function to your theme functions.php that relatively looks for your .git directory and returns your latest commit identifier:
function get_current_git_commit( $branch='master' ) { // you can specify a different branch here if you wish
if ( $hash = file_get_contents( sprintf( '../.git/refs/heads/%s', $branch ) ) ) {
return trim($hash);
} else {
return false;
}
}
Note: you might need to edit the relative path of the file_get_contents to suit where your .git directory is compared to your theme folder.
Then if you enqueue your CSS and JS through your functions.php (like you should be) then you can do it like so…
function register_custom_scripts(){
// get latest commit identifier
$commit = get_current_git_commit();
// enqueue you styles and scripts
wp_enqueue_style( 'custom_styles', get_template_directory_uri().'/dist/css/main.min.css?v='.$commit );
wp_enqueue_script( 'custom_script', get_template_directory_uri().'/dist/js/main.min.js?v='.$commit, array(),'', true);
}
add_action('wp_enqueue_scripts','register_custom_scripts');
Then in the href and src for your CSS/JS output you will see something like the following:
// CSS
https://example.com/dist/css/main.min.css?v=bda6c3e190e61837bcab53bbe4805804ee
// JS
https://example.com/dist/js/main.min.js?v=bda6c3e190e61837bcab53bbe4805804ee